Scoping – where do variables live? – Where can we access a certain variable?
Notes from JS Udemy Course
JS is a high level, object oriented multi paradigm programming language
Lexical scoping – scoping is controlled by placement of functions and blocks in the code
Scope is the space or environment in which a certain variable is declared.
There are 3 main types of scope for variables:
Global scope
Function scope
Block scope
The Scope of a variable, is the region of our code where a certain variable can be accessed.
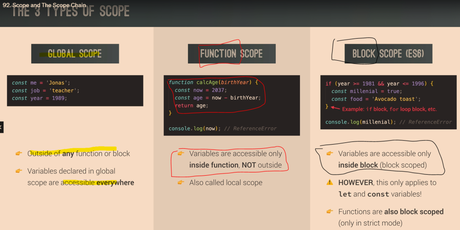
Scope Chain in JavaScript
I asked Chat GPT “what is scope chain in JavaScript?”
A scope chain in JavaScript refers to the hierarchical order of scopes, with each scope having access to its own variables and those of its parent scopes.
This hierarchy is established based on where functions and blocks are physically located within the code (lexical scoping).
When a variable is referenced in JavaScript, the engine looks for the variable in the current scope.
If it doesn’t find it there, it moves up the chain to the parent scope. This process continues all the way up to the global scope if necessary. If the variable isn’t found in the global scope, a ReferenceError is thrown.
Here’s a simple example to demonstrate the concept:
let globalVar = "global"; // global scope
function outer() {
let outerVar = "outer"; // outer function scope
function inner() {
let innerVar = "inner"; // inner function scope
console.log(innerVar); // "inner", found in inner scope
console.log(outerVar); // "outer", not found in inner scope but found in outer scope
console.log(globalVar); // "global", not found in inner or outer scopes but found in global scope
}
inner();
}
outer();
In the above example, the inner
function’s scope chain includes the inner
scope, the outer
scope, and the global scope. The outer
function’s scope chain includes the outer
scope and the global scope. The global scope is at the top of every scope chain.
The scope chain is fundamental to understanding variable lookup and closure behavior in JavaScript.
udemy.com/course/the-complete-javascript-course/learn/lecture/22648487#learning-tools