Notes about primitives and objects data types in JavaScript
I’ve got a bit fed up of the Udemy course I’m doing on JavaScript and the videos, so I’m taking notes from different websites and I’ll do a summary at the end.
Primitive Data Types
Primitive data types are number, string, boolean, NULL, Infinity and symbol. Non-primitive data types is the object. The JavaScript arrays and functions are also objects. For more check out this web developer course online.
What is the main difference between primitive types and objects in JavaScript?
First, let’s define what are primitive types.
Primitive types in JavaScript are
- strings
- numbers (Number and BigInt)
- booleans (true or false)
- undefined
- Symbol values
null
is a special primitive type. If you run typeof null
you’ll get 'object'
back, but it’s actually a primitive type.
Everything that is not a primitive type is an object.
Functions are objects, too. We can set properties and method on functions. typeof
will return 'function'
but the Function constructor derives from the Object constructor.
The big differences between primitive types and objects are
- primitive types are immutable, objects only have an immutable reference, but their value can change over time
- primitive types are passed by value. Objects are passed by reference
- primitive types are copied by value. Objects are copied by reference
- primitive types are compared by value. Objects are compared by reference
Javascript Types
There are eight data types in Javascript:
- string
- number
- bigint
- boolean
- undefined
- null
- symbol
- Object
The first 7 of them are commonly called Primitive Types and everything else are Object Types.
Primitive Types
They can only store a single data, have no methods and are immutable. (An immutable value is one whose content cannot be changed without creating an entirely new value. In JavaScript, primitive values are immutable — once a primitive value is created, it cannot be changed, although the variable that holds it may be reassigned another value).
That’s another interesting point! Primitive Types
have no methods but, except for null
and undefined
, they all have object equivalents that wrap the primitive values then we’re able to use methods.
For string
primitive there is String
object, for number
primitive there is Number
, and so there are Boolean
, BigInt
and Symbol
.
Javascript automatically converts the primitives to their corresponding objects when a method is to be invoked. Javascript wraps the primitive and call the method.
Object Types
Differently from the primitives, Objects can store collections of data, their properties, and are mutable.
Differences between types
1. Assigning to a variable and copying value
The difference in the way the values are stored in variables is what makes people usually call Object Types
as Reference Types
.
Primitive Types
When we assign a primitive type to a variable, we can think of that variable as containing that primitive value.
let car = "tesla" let year = 2021 // Variable - Value // car - "tesla" // year - 2021
So when we assign this variable to another variable, we are copying that value to the new variable. Thus, primitive types are “copied by value”.
let car = "tesla" let newCar = car // Variable - Value // car - "tesla" // newCar - "tesla"
Since we copied the primitive values directly, both variables are separate and if we change one we don’t affect the other.
let car = "tesla" let newCar = car car = "audi" // Variable - Value // car - "audi" // newCar - "tesla"
Object Types
With Object Types
things are different. When we assign an object to a variable, the variable is given a reference to that value. This reference stores the address
to the location of that value in memory(techically more than that but let’s simplify). So the variable doesn’t has the value itself.
Let’s imagine the variable, the value it stores, the address in memory and the object in the coming snippets:
let cars = ["tesla"] // Variable - Value - Address - Object // cars - <#001> (The reference) - #001 - ["tesla"]
This way, when we assign this variable to another one we are giving it the reference for the object and not copying the object itself like it happens with the primitive value. Thus, objects types are “copied by reference”.
let cars = ["tesla"] let newCars = cars // Variable - Value - Address - Object // cars - <#001> (The reference) - #001 - ["tesla"] // newCars - <#001> (The reference stores the same address) cars = ["tesla", "audi"] // Variable - Value - Address - Object // cars - <#001> (The reference) - #001 - ["tesla", "audi"] // newCars - <#001> (The reference stores the same address) console.log(cars) // ["tesla", "audi"] console.log(newCars) // ["tesla", "audi"]
Both of them have references to the same array object. So when we modify the object from one of the variables the other will also have this change.
- Primitives are ummutable
- You can’t alter the original primitive, instead it will return a new primitive value
- Primitives, also have an object counterpart
- When used with some methods, primitive values get wrapped into an object – once it’s finished, it turns back to a primitive value
- It does this, because objects can have properties
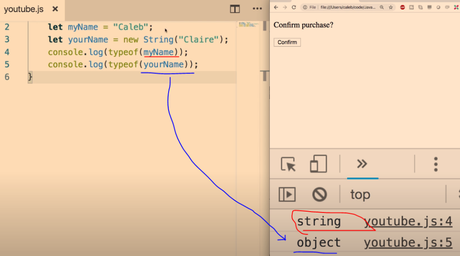
- The new keyword creates a blank object, it links the blank object to the parent