A function is a piece of code, that can be reused.
It’s similar to a variable but doesn’t hold a value like a variable does. So it’s not that much like a variable, but kind of is.
Screenshots are from the brilliant Javascript Course on Udemy here
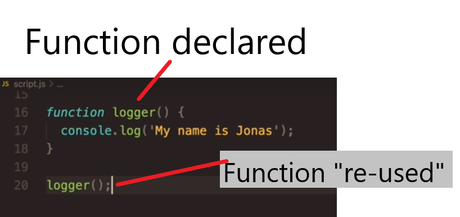
The code on lines 16 and 17 shown in the image above, creates the function. It is named “logger”.
the shorter code logger(); is used to/for “invoke”, “running”, or “calling” the function.
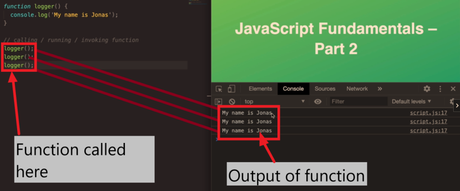
Function Declerations Vs Expressions
Function Declerations
When you create a function with a name, then it is a function decleration.
Function statements, declare a function. A declared function can be used later when it is “invoked” or “called”.
Function declerations must start with “function”.
For example:
function mma() {
return 8;
}
The functioned mma is declared. to invoke it use the code mma();
You can call function declerations, above the code that creates it.
Function Expressions (good article here)
A JavaScript function can also be defined using an expression.
A function expression can be stored in a variable:
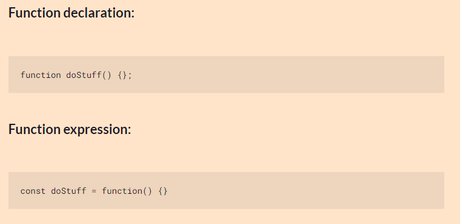
Image source
Function expressions are invoked to avoid polluting the global scope. Instead of your program being aware of many different functions, when you keep them anonymous, they are used and forgotten immediately.
To summarize, function declarations are utilized when you desire to establish a function in the global scope that can be accessed anywhere in your code. On the other hand, function expressions are employed to confine the accessibility of the function, reduce the weight of the global scope, and maintain a neat syntax.
JavaScript Arrow Function
Arrow functions have shorter syntax
They are best suited to non-method functions
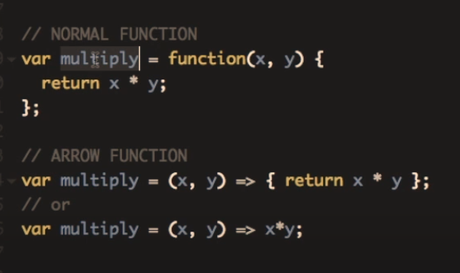
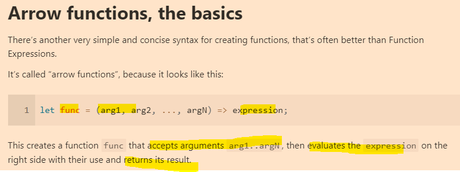
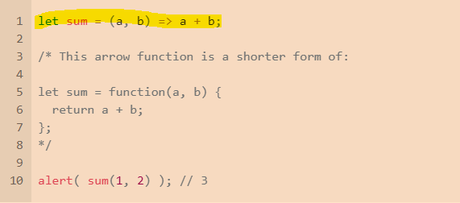
Functions Calling Other Functions
An example, might be a function that cuts up fruit pieces, and then sends the result to a function that blends it into a smoothie.
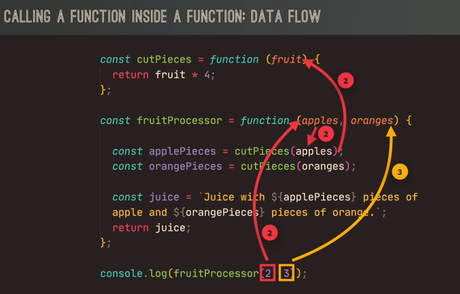
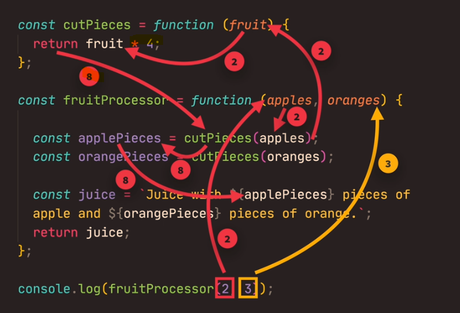
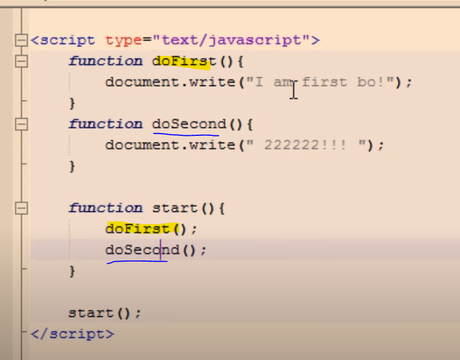
The start function used above, will print out “I am first bo! 222222!!!”
Functions Summary
A function is like a procedure. It has tasks and calculates a value.
A function requires input and must return an output.
Functions need to be defined with the word “function” and brackets ()
Brackets can also be called paranthesies, and the input is sometimes called “arguments” or “parameters”.
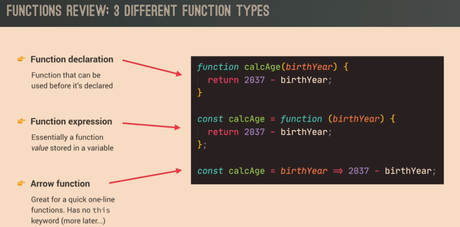
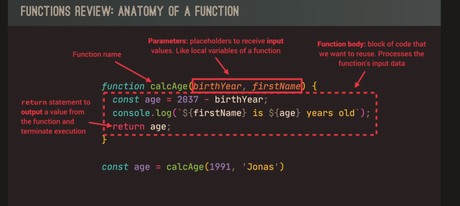
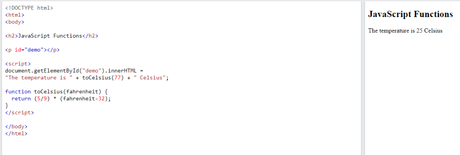