Notes taken from various websites including:
https://www.w3schools.com/js/js_whereto.asp
https://developer.mozilla.org/en-US/docs/Web/JavaScript/Guide/Functions
JavaScript Functions
A JavaScript function
is a block of JavaScript code, that can be executed when “called” for.
For example, a function can be called when an event occurs, like when the user clicks a button.
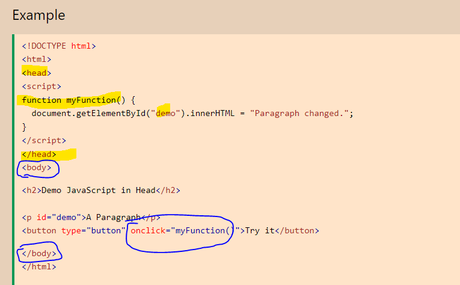
A function in JavaScript is similar to a procedure—a set of statements that performs a task or calculates a value, but for a procedure to qualify as a function, it should take some input and return an output where there is some obvious relationship between the input and the output.
Function declarations
A function definition (also called a function declaration, or function statement) consists of the function keyword, followed by:
The name of the function.
A list of parameters to the function, enclosed in parentheses and separated by commas.
The JavaScript statements that define the function, enclosed in curly brackets, { /* … */ }.
For example, the following code defines a simple function named square:
function square(number) {
return number * number;
}
The function square takes one parameter, called number. The function consists of one statement that says to return the parameter of the function (that is, number) multiplied by itself. The statement return specifies the value returned by the function:
return number * number;
Parameters are essentially passed to functions by value — so if the code within the body of a function assigns a completely new value to a parameter that was passed to the function, the change is not reflected globally or in the code which called that function.
When you pass an object as a parameter, if the function changes the object’s properties, that change is visible outside the function, as shown in the following example:
function myFunc(theObject) {
theObject.make = “Toyota”;
}
const mycar = {
make: “Honda”,
model: “Accord”,
year: 1998,
};
console.log(mycar.make); // “Honda”
myFunc(mycar);
console.log(mycar.make); // “Toyota”
Differences between functions and methods (taken from Geeks for Geeks)
A JavaScript function is a block of code designed to perform a particular task.The javascript method is an object property that has a function value.
Syntax of Function is -:function functionName(parameters) {
// Content
}Syntax of Method is -:object = {
methodName: function() {
// Content
}
};object.methodName()
A function can pass the data that is operated and may return the data. The method operates the data contained in a Class.
Data passed to a function is explicit.A method implicitly passes the object on which it was called.
A function lives on its own.A method is a function associated with an object property.
A function can be called directly by its name A method consists of a code that can be called by the name of its object and its method name using dot notation or square bracket notation.
Functions are helpful because it increases the reusability of the code.Javascript includes some in-built methods also for example -: parseInt() Method
The () Operator is used to Invoke the FunctionWe can access object method by the following the syntax -:objectName.methodName()
External JavaScript Advantages
Javascript can also be placed in the <body> of a HTML document and in external sheets with the file extension .js (not sure why I thought this was relevant, but there you go!)
Placing scripts in external files has some advantages:
It separates HTML and code
It makes HTML and JavaScript easier to read and maintain
Cached JavaScript files can speed up page loads
JavaScript Output
JavaScript can interact with the DOM/webpage and display different data in several ways:
- Wrting into an HTML element using innerHTML
- Wirting into the HTML output using document.write()
- Writing into an alert box, using window.alert()
- Wiring into the browser console, using console.log()
Using InnerHTML
use the document.getElementByID(id) method.
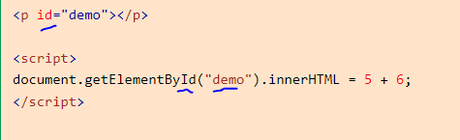
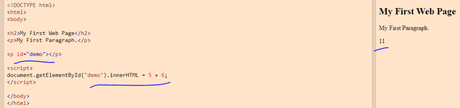
JavaScript Events
HTML events are “things” that happen to HTML elements.
When JavaScript is used in HTML pages, JavaScript can “react” on these events.
more info at w3schools
JavaScript’s interaction with HTML is handled through events that occur when the user or the browser manipulates a page.
When the page loads, it is called an event. When the user clicks a button, that click too is an event. Other examples include events like pressing any key, closing a window, resizing a window, etc.
Developers can use these events to execute JavaScript coded responses, which cause buttons to close windows, messages to be displayed to users, data to be validated, and virtually any other type of response imaginable.
Events are a part of the Document Object Model (DOM) Level 3 and every HTML element contains a set of events which can trigger JavaScript Code.
Please go through this small tutorial for a better understanding HTML Event Reference. Here we will see a few examples to understand a relation between Event and JavaScript −
onclick Event Type
This is the most frequently used event type which occurs when a user clicks the left button of his mouse. You can put your validation, warning etc., against this event type.
Example
Try the following example.
<html> <head> <script type = "text/javascript"> <!-- function sayHello() { alert("Hello World") } //--> </script> </head> <body> <p>Click the following button and see result</p> <form> <input type = "button" onclick = "sayHello()" value = "Say Hello" /> </form> </body> </html>
More info at Tutorialspoint (includes a massive list of event types)