Software patterns, often referred to as design patterns, are a reusable and generalizable solution to a common problem that software developers face when designing and building software applications. Design patterns are essentially templates or blueprints for solving recurring design problems in a systematic and efficient way.
Design patterns serve several important purposes in software development:
- Reusability: They encapsulate proven solutions to common problems, making it easier for developers to reuse these solutions in different parts of their code or in different projects.
- Abstraction: Design patterns provide a higher-level abstraction that helps developers think about and communicate the structure of their code more effectively. They provide a common language and framework for discussing design decisions.
- Maintainability: Using design patterns can improve the maintainability of software because they promote well-structured and organized code. Developers can more easily understand and modify code that follows established patterns.
- Scalability: Patterns can help ensure that software is designed in a way that allows it to scale and adapt to changing requirements without requiring major redesigns.
- Community Knowledge: Design patterns are well-documented and widely recognized within the software development community. This means that developers can leverage the collective wisdom and experience of the community when applying these patterns.
Photo by Kevin Ku on Pexels.com
" data-medium-file="https://i0.wp.com/francescolelli.info/wp-content/uploads/2023/10/pexels-photo-577585.jpeg?fit=300%2C225&ssl=1" data-large-file="https://i0.wp.com/francescolelli.info/wp-content/uploads/2023/10/pexels-photo-577585.jpeg?fit=790%2C593&ssl=1" src="https://i0.wp.com/francescolelli.info/wp-content/uploads/2023/10/pexels-photo-577585.jpeg?resize=790%2C592&ssl=1" alt="What is a Software Patterns or a Design Patterns and an introduction to the most common one" class="wp-image-2518" srcset="https://i0.wp.com/francescolelli.info/wp-content/uploads/2023/10/pexels-photo-577585.jpeg?w=1734&ssl=1 1734w, https://i0.wp.com/francescolelli.info/wp-content/uploads/2023/10/pexels-photo-577585.jpeg?resize=300%2C225&ssl=1 300w, https://i0.wp.com/francescolelli.info/wp-content/uploads/2023/10/pexels-photo-577585.jpeg?resize=1024%2C768&ssl=1 1024w, https://i0.wp.com/francescolelli.info/wp-content/uploads/2023/10/pexels-photo-577585.jpeg?resize=768%2C576&ssl=1 768w, https://i0.wp.com/francescolelli.info/wp-content/uploads/2023/10/pexels-photo-577585.jpeg?resize=600%2C450&ssl=1 600w, https://i0.wp.com/francescolelli.info/wp-content/uploads/2023/10/pexels-photo-577585.jpeg?resize=1536%2C1152&ssl=1 1536w" sizes="(max-width: 790px) 100vw, 790px" data-recalc-dims="1" />What are Software Patterns (or Design Patterns) and an introduction to the most common oneAs the term software embrace a very large domain, there are several categories of design patterns. Each one is specialized for a particular portion portion of the code. These patterns includes:
- Creational Patterns: These patterns deal with object creation mechanisms, trying to create objects in a manner suitable to the situation. Examples include the Singleton, Factory Method, and Abstract Factory patterns.
- Structural Patterns: Structural patterns focus on defining the composition of classes and objects. Examples include the Adapter, Bridge, and Decorator patterns.
- Behavioral Patterns: Behavioral patterns are concerned with the interaction and communication between objects. Examples include the Observer, Strategy, and Command patterns.
- Architectural Patterns: These are high-level patterns that deal with the overall structure of an application. Examples include the Model-View-Controller (MVC), Model-View-ViewModel (MVVM), and Layered Architecture patterns.
- Concurrency Patterns: These patterns address issues related to managing concurrent access to shared resources in multi-threaded applications. Examples include the Mutex, Semaphore, and Read-Write Lock patterns.
Design patterns are not one-size-fits-all solutions, and they should be applied judiciously based on the specific requirements and constraints of a software project. Experienced developers use their knowledge of design patterns to make informed decisions about when and how to apply them to solve particular problems.
The list of pattern is long and is also evolving as the software developer community evolve. At the Some time pattern like facade, adapter/wrapper, proxy and observer stand the test of time and are the most known among developers. Let’s see each one of them
Facade Pattern:
- Purpose: The Facade pattern provides a simplified, high-level interface to a complex subsystem or set of classes. It acts as a “facade” to shield clients from the underlying complexities of the system.
- Use Cases: It’s useful when you want to provide a simplified and unified interface to a complex system, making it easier for clients to interact with it. It promotes loose coupling between the client code and the subsystem.
The image below present the pattern using a Class Diagram:
Example of Use: Multimedia Player Facade
Suppose you are building a multimedia player application that can play various types of media, such as audio and video files. The underlying multimedia framework is complex, with different classes and interfaces for handling codecs, rendering, audio output, and video display.
In this example, we could consider implementing a class of name MultimediaPlayerFacade
that acts as a simplified interface to the complex multimedia framework. It encapsulates the initialization and usage details of the audio and video players, making it much easier for client code to play multimedia. The client code is simplified and less coupled to the underlying multimedia framework, which can change without affecting the client code.
The Facade pattern here provides a cleaner and more maintainable way to interact with a complex subsystem, shielding the client code from its intricacies.
Adapter Pattern also known as Wrapper Pattern::
- Purpose: The Adapter pattern allows two incompatible interfaces to work together by providing a wrapper around one of them. It acts as a bridge between two interfaces, making them compatible without changing their source code.
- Use Cases: It’s used when you have existing code with interfaces that are not compatible with the interfaces you need to use. The adapter pattern allows you to reuse existing code without modification.
The image below present the pattern using a Class Diagram:
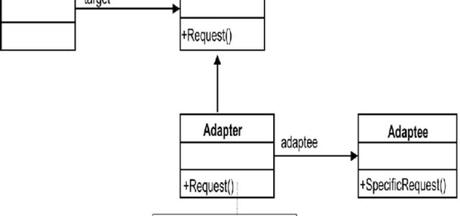
Example of use: Legacy System Integration
Imagine you are working on a modern e-commerce application that needs to integrate with a legacy payment gateway. The legacy payment gateway has an outdated interface that doesn’t match the modern payment service interface your application uses. You can use the Adapter pattern to bridge the gap.
To integrate the legacy payment gateway with your modern payment service, you can create an adapter class. In this way you can use the Legacy Payment Adapter
to make payments through the legacy payment gateway using the modern payment service. In this way the adapter acts as a bridge between the modern payment service interface and the legacy payment gateway.
Proxy Pattern:
- Purpose: The Proxy pattern provides a surrogate or placeholder for another object to control access to it. It can be used to add an additional layer of control over an object, such as lazy loading, access control, or caching.
- Use Cases: It’s useful when you want to control access to an object, especially in situations where the creation or access to the object is expensive or where you need to add functionality around object access.
The image below present the pattern using a Class Diagram:
Example of use: Image Loading Proxy
Suppose you are building an image viewer application, and you want to implement a feature that loads and displays high-resolution images from a remote server. However, loading these high-resolution images can be slow and resource-intensive. To improve user experience and optimize resource usage, you can use the Proxy pattern. You can use the ImageProxy
to load and display images in your application without loading the high-resolution image until it’s actually needed. Consequently you can delay the creation and loading of resource-intensive objects, improving the performance and resource utilization of your application.
Observer Pattern:
- Purpose: The Observer pattern defines a one-to-many dependency between objects so that when one object changes state, all its dependents (observers) are notified and updated automatically.
- Use Cases: It’s commonly used for implementing distributed event handling systems, where multiple objects need to react to changes in another object’s state. Examples include GUI frameworks where UI elements update when underlying data changes.
The image below present the pattern using a Class Diagram:
Example of use: Weather Monitoring System
Imagine you are building a weather monitoring system that collects weather data from various sensors and notifies multiple display devices when the weather conditions change. In this scenario, you can apply the Observer pattern to implement the system. You could imagine a class of name ConcreteWeatherStation
as the subject that collects weather data and notifies its observers (in this case, maybe various display objects) when the data changes. The Observer pattern allows multiple display devices to receive and react to updates without being tightly coupled to the weather station.
Summary of Software Patterns or Design patterns
Software patterns, also known as design patterns, are widely recognized and essential approaches for organizing code and solving recurring design problems in software development. Among these patterns, the Facade pattern simplifies complex subsystems by providing a unified interface, making it easier for clients to interact with intricate systems. The Adapter pattern bridges the gap between incompatible interfaces, allowing different components to work seamlessly together. The Proxy pattern acts as a surrogate for an object, controlling access and adding functionality, which can be valuable for resource optimization and security. Lastly, the Observer pattern facilitates the creation of distributed event handling systems, enabling one object to notify multiple observers about changes, enhancing code modularity and flexibility. These patterns represent just a subset of the many design patterns available, and they serve as valuable tools in the developer’s toolkit for building maintainable, scalable, and efficient software systems.
In the case you would like to learn more I would recommend you to have a look at the following book from “the gang of 4“
It is a classic and several libraries will probably have a copy.