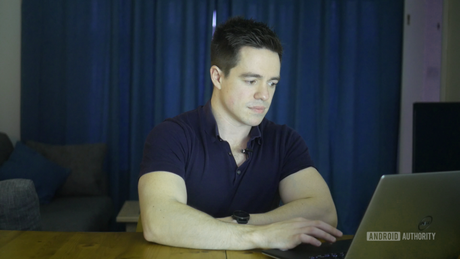
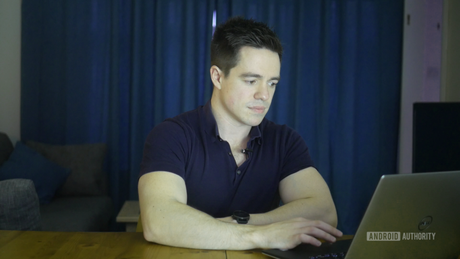
One of the more complicated concepts for finding your way around as a new programmer is classes and objects. However, once you understand how classes are used in Python, you can create much more powerful and complex code.
Also read: What is Object Oriented Programming?
Read on to learn how to use classes in Python, and when you should!
Introduction to classes in Python
For those unfamiliar with the concept of teaching and wanting to learn more about how they work, read on. If you just want the syntax for classes in Python, you can skip to the next section!
What is a class? A class is code that describes a "data object". This is an object as you find it in the real world, only that it has no tangible presence: it only exists in concept!
Like real objects, data objects can have properties (size, weight, size, number of lives, speed) and functions (move forward, jump, increase heat, erase).
In a computer game, for example, a villain could be described in code as a data object. This would keep track of how much health the bad guy had, where it was in relation to the player, and how aggressive it would be. We could then call an Evil One's "shoot" function to fire projectiles, or their "destroy" function to remove them from the game.
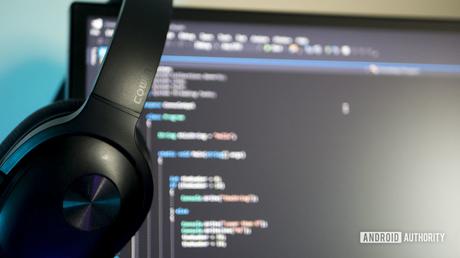
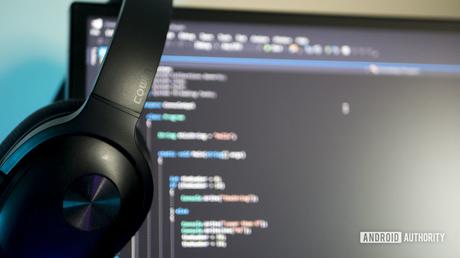
(Except we call functions "methods" when they appear in classes in Python!)
You would then simply use your graphics routines to drag these bad guys onto the screen based on the information provided by the class.
When are Python classes used?
Knowing how variables are used in Python works in a similar way: instead of storing a data item as an integer, you store custom information about an object you design.
Also read: Using strings in Python
The great thing about classes in Python is that they can create multiple "instances" of a single thing. That said, we just need to write a "BadGuy" class to create as many individual bad guys as possible!
What else could you use classes in Python for? A class can be used to describe a particular tool within a program, e.g. B. a score manager, or it can be used to describe entries in a client database. Anytime you want to create many examples of the same "thing", or when you want to export complex code in a modular and easy way, classes are a good choice.
Using classes in Python
Now that you know what to do with classes, you may be wondering how to actually use classes in Python.
Getting started is relatively easy, I have to love Python! You create a class just like you create a function, except you use "class" instead of "def". We'll then name the class, add a colon, and indent whatever follows.
(Note that classes should use capital letters to distinguish them from variables and functions. This means "BadGuy", not "badGuy" or "bad_guy".)
Also read: How to define a function python
So if we wanted to create a class that would represent an enemy in a computer game, it could look like this:
class BadGuy: health = 5 speed = 2
This villain has two properties (variables) that describe his health and how quickly he moves. Outside of this class, we then need to create a BadGuy object before we can access these properties:
bad_guy_one = BadGuy() print(bad_guy_one.health) print(bad_guy_one.speed)
Note that we could just as easily create a bad_guy_two and a bad_guy_three and then display each of their properties!
bad_guy_one = BadGuy() bad_guy_two = BadGuy() print(bad_guy_one.health) print(bad_guy_two.health) bad_guy_one.health -= 1 print(bad_guy_one.health) print(bad_guy_two.health)
Here we have changed the value of the health of one evil but not the other! We edited one example of evil.
Understand instances
To really take advantage of the power of classes in Python, we need to understand Instances and Constructors. If you create two bad guys from the same BadGuy class, each of them is an "instance".
Ideally, we might want to create two bad guys with different initial health. In addition, we might want to change this health from within the BadGuy class.
To do this, we need a special type of method (function in a class) called a "constructor".
The constructor is called whenever you create a new instance of an object (when you "instantiate" the object) and is mainly used to define the variables related to it Specific Instance of the object. Of course, you can also do other things here: for example, send welcome messages.
So for example:
class BadGuy: def __init__(self, health, speed): print("A new badguy has been created!") self.health = health self.speed = speed bad_guy_one = BadGuy(5, 2) bad_guy_two = BadGuy(3, 5) print(bad_guy_one.health) print(bad_guy_two.health)
This code creates two bad guys. One is strong but slow (Health 5, Speed 2), the other is weak but fast (3, 5). Every time a new villain is created, a message will appear telling us that this has happened.
The constructor method is always called __drin__ and will always have "self" as the first argument. You can then pass in any other arguments that you want to use to set up your object the first time it is initialized.
The term "self" simply means that everything you do relates to it Specific Instance of the object.
Using functions in classes in Python
As mentioned earlier, in Python a function is technically called a method.
We can create methods within a class just as we normally create functions, but there are two different types of methods:
An instance method only affects the instance of the object to which it belongs. Hence, we can use this as a more convenient way to deal damage to individual enemies:
class BadGuy: def __init__(self, health, speed): print("A new badguy has been created!") self.health = health self.speed = speed def shoot_badguy(self): self.health -= 1 print("Ouch!") bad_guy_one = BadGuy(5, 2) bad_guy_two = BadGuy(3, 5) def display_health(): print(bad_guy_one.health) print(bad_guy_two.health) display_health() bad_guy_one.shoot_badguy() display_health()
A static method, on the other hand, is designed to act globally. To create static methods, we remove the "self" argument and use the instead @staticmethod Decorator just above the method name.
In the example below, we'll create a static method to generate a random number and subtract that amount from the enemy's health. The method doesn't have to specifically refer to the instance of that object, so it can simply behave like a normal function that we can access when using the class.
class BadGuy: def __init__(self, health, speed): print("A new badguy has been created!") self.health = health self.speed = speed @staticmethod def random_generator(): import random n = random.randint(1, 5) return n def shoot_badguy(self): self.health -= self.random_generator() print("Ouch!") bad_guy_one = BadGuy(5, 2) bad_guy_two = BadGuy(3, 5) def display_health(): print(bad_guy_one.health) print(bad_guy_two.health) display_health() bad_guy_one.shoot_badguy() display_health()
Note that at any point in our code we can also use the following line to get a random number:
print(bad_guy_two.random_generator())
If for any reason we want to prevent this, all we have to do is prefix our method name with a double underscore.
@staticmethod def __random_generator():
That way, you create a private method in Python and you cannot access the method outside of that class.
Conclude
The last thing you may want to do is put your class in a separate file. That way, your code stays tidy and you can easily share the classes you create between projects.
To do this, just save the class in a new file:
class BadGuy: def __init__(self, health, speed): print("A new badguy has been created!") self.health = health self.speed = speed @staticmethod def __random_generator(): import random n = random.randint(1, 5) return n def shoot_badguy(self): self.health -= self.__random_generator() print("Ouch!")
Make sure the file has the same name as the class. In this case: "BadGuy.py" is the name of the file. It also needs to be saved in the same directory where you save your main Python file.
Now you can access the class and all of its properties and methods from any other Python script:
import BadGuy bad_guy_one = BadGuy.BadGuy(5, 2) bad_guy_two = BadGuy.BadGuy(3, 5) def display_health(): print(bad_guy_one.health) print(bad_guy_two.health) display_health() bad_guy_one.shoot_badguy() display_health()
And there you have it! How To Use Classes In Python! This is an extremely valuable skill that will allow you to build all kinds of amazing things in the future.
At this point, you are probably ready to take your skills to the next level. In that case, try one of these amazing online Python courses:
Coding with Python: The Budding Developer Training course provides you with a comprehensive introduction to Python that takes you from the basics of coding to highly-skilled skills that prepare you for a career in Python development. This course typically costs $ 690 but is available Android Authority Readers for only $ 49!
Alternatively, check out our comprehensive beginner's guide to Python to see how classes fit into the bigger picture:
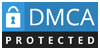