<dependency> <groupId>org.jboss.resteasy</groupId> <artifactId>resteasy-jaxrs</artifactId> <version>2.3.2.Final</version> <scope>provided</scope> </dependency>Now create a root rest service. By default it returns Empty set of classes in getClasses method which means whole web Application will be scanned to find jax rs annotations.
package rest; import java.util.Set; import javax.ws.rs.core.Application; public class RootRestService extends Application{ public Set> getClasses() { return super.getClasses(); } }This is our sample Rest Class .
package rest; import java.io.IOException; import java.util.HashMap; import java.util.Map; import javax.ws.rs.Consumes; import javax.ws.rs.GET; import javax.ws.rs.POST; import javax.ws.rs.Path; import javax.ws.rs.Produces; import javax.ws.rs.core.MediaType; import javax.ws.rs.core.Response; import javax.ws.rs.core.Response.Status; @Path("/application") public class RestService { HttpClient cl = new HttpClient(); @Path("/test") @GET @Produces(MediaType.TEXT_PLAIN) public Response test() { return Response.status(Status.OK).entity("working").build(); } }Now in your web.xml , you have to define a servlet to dispatch your request to rest service. Provide url pattern for the dispatcher. in our case it is /rest/* , accordingly you have to define the prefix in context parameter . So if the url pattern is /rest/* then prefix will also be /rest.If you map the dispatcher to /* pattern , then you will not be able to access other resources in your web app like html ,css,jsp ,servlets etc . because every request will go through the rest easy dispatcher and you will get 404 error .So ,it's better to have a separate prefix for rest services in web app.
<context-param> <param-name>resteasy.servlet.mapping.prefix</param-name> <param-value>/rest</param-value> </context-param> <context-param> <param-name>resteasy.resources</param-name> <param-value>rest.RestService</param-value> </context-param> <servlet> <servlet-name>resteasy-servlet</servlet-name> <servlet-class> org.jboss.resteasy.plugins.server.servlet.HttpServletDispatcher </servlet-class> <init-param> <param-name>javax.ws.rs.Application</param-name> <param-value>rest.RootRestService</param-value> </init-param> </servlet>You may get error like this : Could not find resource for relative : /application/test of full path: http://localhost:8080/onlinehttpclient/rest/application/test To resolve this error , you have to define resteasy.resource context param with the full path of Rest class.
Post Comments And Suggestions !!
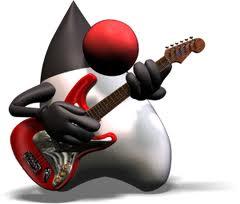