Conditional statements in c
The conditional statements in c are the statements that are used to check any particular condition according to the user’s requirement. These conditional statements in c help us to make a decision in the program in any condition.
We specify the condition as a set of the conditional statements having the Boolean expression which may be either true or false. Execution flow of a c program is changed as per the condition specified in conditional statements.
Different types of conditional statements in c
In C programming language there are following types of conditional statements in c.
1. if statement
2.if-else statement
3.nested if else statement
4.switch statement
5.if else if ladder
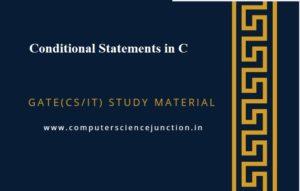
Let us see these conditional statements in C one by one
1.if statement in c
When if is used as a single statement in C program then code inside the if block will execute if a condition is true. It is also called one-way selection statement.
The general syntax of if statement in c is given below
if(expression)
{
//code to be executed
}
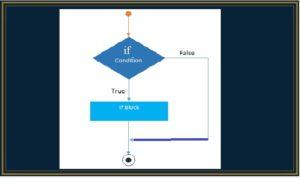
If the condition is evaluated as true then the block statement is executed but if the condition is evaluated as false then control is passed to the next statement following it.
if statement in c Example
#include
#include
void main()
{
int n;
printf(“enter the number”);
scanf(“%d”,&n);
if(n%2==0)
{
printf(“%d number in even”,n);
}
getch();
}
2. if else statement in c
if else statement in c allows two-way selection. If the given condition is true. If the condition is true then program control goes inside the if block and execute the statement.
The condition is false then program control goes inside the else block and execute the corresponding statement.
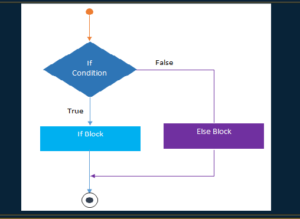
The general syntax of if else statement in c is given as following.
if(expression or condition)
{
// statement ;
}
else
{
//statement ;
}
if-else statement Example
#include
#include
void main()
{
int n;
printf(“enter the number”);
scanf(“%d”,&n);
if(n%2==0)
{
printf(“%d number in even”,n);
}
else
{
Printf(“%d number is odd”,n);
}
getch();
}
3. nested if else Statement
When we need to test more than one expression then we use nested if else statement. Nested if else is also known as multi-way selection statement. If there is a series of decision is involved in a statement we use if else in nested form.
The general syntax of else if-else-if is as follows.
if(expression)
{
Statement
}
else if
{
// Statement
}
else if
{
//Statement
}
else
{
// Statement
}
The execution of the above nested if-else statement is as follows
Firstly it will check the given condition if the result of the given condition is True then the statements related, if the given condition is false then it will check the else if part and the condition of is true then the statements related to else if is Executed otherwise the pointer goes to next else if and this process is contained And if the result of the all the else if condition is false then the pointer is automatically going to else part and the statement of the else automatically executed.
nested if-else Example
Following the program to find the greatest number among the three number.
#include
#include
void main( )
{
int a,b,c;
clrscr();
printf(“Please Enter three number”);
scanf(“%d%d%d”,&a,&b,&c);
if(a>b)
{
if(a>c)
{
printf(“a is greatest number”);
}
else
{
printf(“c is greatest number”);
}
}
else
{
if(b>c)
{
printf(“b is greatest number”);
}
else
{
printf(“c is greatest”);
}
}
getch();
}
4. if-else-if ladder
The if-else-if conditional statement in c is used to execute one code from multiple conditions. It is also known as multi path decision statement. It is a sequence of if..else statements where every if a statement is associated with else if statement and last would be an else statement.
The general syntax of if – else- if ladder is shown below
if(expression1)
{
//statements
}
else if(expression2)
{
//statements
}
else if(expression3)
{
//statements
}
else
{
//statements
}
if else if ladder example
#include
#include
void main( )
{
int a;
printf(“enter a number”);
scanf(“%d”,&a);
if( a%5==0 & a%8==0)
{
printf(“This number divisible by both 5 and 8”);
}
else if( a%8==0 )
{
printf(“This number is divisible by 8”);
}
else if(a%5==0)
{
printf(“this number is divisible by 5”);
}
else
{
printf(“This number is divisible by neither 8 nor 5”);
}
getch();
}
5 . Switch Case Statement
A switch case statement in C Programming tests the value of a choice variable and compares it with multiple cases. When the case match is found, a block of statements associated with that specific case is executed.
Each case in a block of a switch has a different choice which is referred to as an identifier. When the user enter a value then the value provided by the user is compared with all the cases inside the switch block until the exact match is found.
If a case match is not found, then the default statement is executed, and the control goes out of the switch block.
switch case statement example
Program to demonstrate the use of switch statement is as follow
#include
#include
void main()
{
int choice;
printf( “Enter any valuen” );
scanf( “%d”, & choice );
switch ( choice ) {
case 1:
printf( “It is hot weather!n” );
break;
case 2:
printf( “It is a stormy weather!n” );
break;
case 3:
printf( “It is a sticky weather!n” );
break;
default:
printf( “It is a pleasant weather!n” );
break;
}
getch();
}