I asked chat GPT to explain the Nullish coalescing operator (??) to me, like I’m 10 years old:
Let’s imagine you have two boxes, one is called ‘boxA’ and the other is ‘boxB’.
Now, you’re told you can take a toy from boxA, but here’s the thing, sometimes boxA can be empty. If boxA is empty, you’re allowed to take a toy from boxB instead.
In JavaScript, this is what the nullish coalescing operator (??) does. If the value on its left side (boxA) is “null” or “undefined” (it’s empty), it’ll give you the value on the right side (boxB).
Let’s look at this in code:
let boxA = null;
let boxB = "Dinosaur Toy";
let myToy = boxA ?? boxB;
console.log(myToy); // This will print: Dinosaur Toy
In this example, ‘boxA’ is null (it’s empty), so we move to ‘boxB’ and take the “Dinosaur Toy”.
But what if ‘boxA’ does have a toy?
let boxA = "Robot Toy";
let boxB = "Dinosaur Toy";
let myToy = boxA ?? boxB;
console.log(myToy); // This will print: Robot Toy
Here, ‘boxA’ has a “Robot Toy”, so we take that and ignore what’s in ‘boxB’. It’s just like the rule we set at the start, we only check ‘boxB’ if ‘boxA’ is empty!
I hope that helps explain the nullish coalescing operator!
Notes from this video^
This operator works like the logical “or”, but is more strict
In this scenario, at the start of a game, the user has to provide the level of difficulty they want, and they also have the option of stating/providing at starting cash amount.
In this code, if they don’t provide a starting cash amount, they’ll get “500”
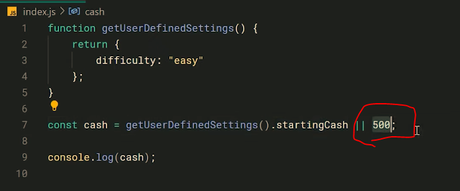
So the default for the “cash” is 500
If the user does provide a cash amount, then that gets used instead of the default.
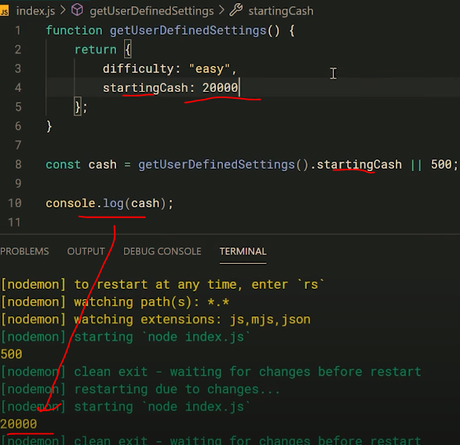